Avectra.netForum.Data.iWebControlClass
The Avectra.netForum.Data.iWebControlClass is an inheritable Class that can be used to develop Visual Studio .NET User Controls in iWeb.
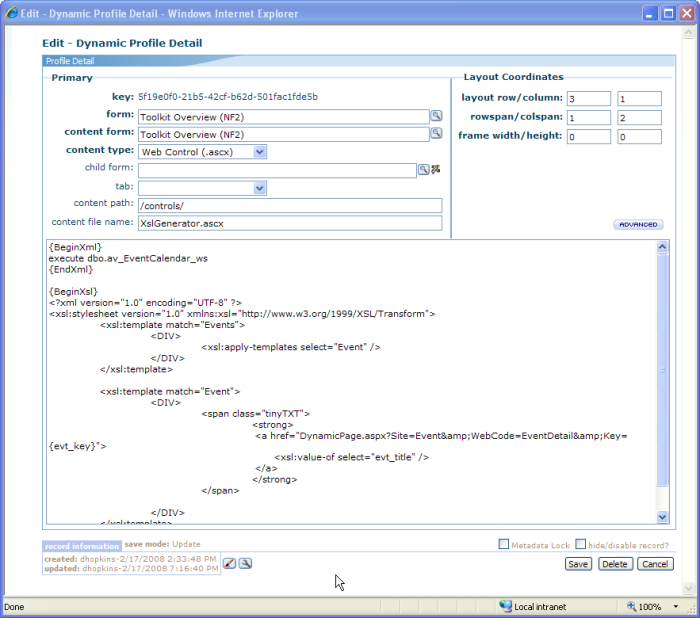
Unlike an ordinary iWeb User Control, the iWebControlClass allows you to get access to the Profile Detail metadata in particular the content data (the large textarea, see nearby image). This allows you to develop a Control in which the code can get information from the content data. You might have one generic control that acts differently depending on the context and depending on the metadata in the Profile Detail.
Properties
- szData (string). The Content Data as entered in the Profile Detail.
- szPath (string). The Content Path of the Profile Detail.
- szPath (string). szDetailKey
- oFacadeObject (FacadeClass). The FacadeObject of the page.
- DesignLinkTable (HtmlTable). A HtmlTable that contains a design link pointing to the Profile Detail. You should add this HtmlTable to the Controls collection so admin users will have the design link.
Methods
GetListProperty
public string GetListProperty(ref string szHtml, string szBeginTag)
Returns any data between the {BeginXXX} and {EndXXX} where XXX is what is passed in the szBeginTag property. This method can be used to extract custom-defined metadata from the content data of the Profile Detail.
For example, if you have this in the content data:
{BeginParam}{CustomerKey}{EndParam}
Then here is how you can extract it:
///This will return the "parsed value" of {CustomerKey}
string szContentData = this.szData;
string szcst_key = this.GetListProperty(szContentData, "Param");
/// szcst_key will be "abcd-1234" if this is what the {CustomerKey} is
For this content data:
{BeginWrite}hello{EndWrite}}
This code will extract it:
string szContentData = this.szData;
string sztext = this.GetListProperty(ref szContentData , "Write");
/// sztext will be "hello"
GetListProperty Overloads
public string GetListProperty(ref string szHtml, string szBeginTag, bool bParseValues)
By default, GetListProperty will parse values. If you do not want values to be parsed, then pass false in the bParseValues parameter.
public string GetListProperty(ref string szHtml, string szBeginTag, bool bParseValues, bool bHtmlDecode)
By default, GetListProperty will HtmlDecode. If you don't want this, then pass false to the bHtmlDecode parameter.
Usage and Examples
Here is a sample User Control that inherits from iWebControlClass. You must add a reference to Data.dll.
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using Avectra.netForum.Data;
namespace AUDC2008iWeb.controls
{
public partial class AUDCUserControl : Avectra.netForum.Data.iWebControlClass
{
protected void Page_Load(object sender, EventArgs e)
{
// Get the Content Data
string szContentData = this.szData;
// Get the Content Path
string szPath = this.szPath;
// Call GetListProperty method to get value between {BeginWrite}{EndWrite}
// in the Content Data of the "profile detail" metadata
string szText = this.GetListProperty(ref szContentData , "Write", true);
// Get the parent PageClass if needed
Avectra.netForum.Data.PageClass oPage = ((Avectra.netForum.Data.PageClass)this.Page);
// Get a value from the FacadeObject
string szText = this.oFacadeObject.GetValue("zzz_key");
// Create a control to output
Label oLabel = new Label();
oLabel.Text = szText;
// Add control to collection so it will output
this.Controls.Add(oLabel);
// Add the design link:
this.Controls.Add(this.DesignLinkTable);
}
}
}