xWeb: ExecuteMethod Advanced C#
This topic shows you how to develop a new ASP.NET Web Service Application that inherits from xWeb and employs the ExecuteMethod web method.
Classes Library
First, create a Class Library project called AUDC2008Web with references to xWebClasses.dll.
Add this class called EventDetail_ExecuteMethod.cs.
This develops classes and a web method with a return powered by the DynamicXmlBuilder, which is the NetForum class that powers ExecuteMethod internally.
using System;
using System.Collections.Generic;
using System.Text;
using System.Xml;
using System.Xml.Serialization;
using Avectra.netForum.xWeb;
using Avectra.netForum.xWeb.xWebSecure;
namespace AUDC2008WSClasses
{
class EventDetail_ExecuteMethod
{
}
#region Events Classes
[Serializable]
public class EventList
{
public EventList()
{}
public Events Events = new Events();
}
public class Events : System.Collections.CollectionBase
{
public Events()
{ }
public Event this[int nIndex]
{
get
{
return (Event)this.InnerList[nIndex];
}
}
/// <summary>
/// Add a single Detail to the collection
/// </summary>
/// <param name="oParameter"></param>
public void Add(Event oParameter)
{
this.List.Add(oParameter);
}
}
[Serializable]
public class Event
{
public Event()
{ }
public string evt_key;
public string evt_title;
public DateTime evt_start_date;
public DateTime evt_end_date;
public string evt_etp_key;
public Sessions Sessions = new Sessions();
}
[Serializable]
public class Session
{
public Session()
{ }
public string ses_key;
public string ses_title;
}
[Serializable]
public class Sessions : System.Collections.CollectionBase
{
public Sessions()
{}
public Session this[int nIndex]
{
get
{
return (Session)this.InnerList[nIndex];
}
}
/// <summary>
/// Add a single Detail to the collection
/// </summary>
/// <param name="oParameter"></param>
public void Add(Session oParameter)
{
this.List.Add(oParameter);
}
}
#endregion
public struct Functions
{
/// <summary>
/// Returns EventList based on specified Event Key
/// </summary>
/// <param name="evt_key">Event Key</param>
/// <param name="userName">xWeb User Name for Authorization</param>
/// <returns></returns>
public static EventList EventDetail(string evt_key, string userName)
{
EventList EventList = new EventList();
/// Call DynamicXmlBuilder, which is the Class that powers ExecuteMethod
Avectra.netForum.xWeb.DynamicXmlBuilder xmlbuilder = new DynamicXmlBuilder();
/// Set the WebServiceName and WebMethod methods based on
/// Web Method setup in iWeb
xmlbuilder.WebServiceName = "AVEventInformation";
xmlbuilder.WebMethod = "EventDetail";
// Add as many parameters as needed
xmlbuilder.Parameters.Add("evt_key", evt_key);
// Validate based on the xWeb User that authenticated
xmlbuilder.ServerIP = userName;
XmlNode oNode;
try
{
oNode = xmlbuilder.BuildDynamicXmlNode();
}
catch (Exception ex)
{
throw new Exception("Error:" + ex.Message);
}
if (oNode != null)
{
// Deserialize XmlNode into Classes
try
{
XmlSerializer serializer = new XmlSerializer(typeof(EventList));
System.Xml.XmlNodeReader oReader = new System.Xml.XmlNodeReader(oNode);
EventList = (EventList)serializer.Deserialize(oReader);
}
catch (Exception ex)
{
throw new Exception("Error:" + ex.Message);
}
}
return EventList;
}
}
}
If you want to squeeze more performance, you can execute the SQL in your code instead of in DynamicXmlBuilder. Note that this approach does not implement any security restrictions, other than validating the token. Please note that this code is a sample and has not been tested.
public static EventList EventDetail(string evt_key, string userName)
{
EventList EventList = new EventList();
SqlConnection connection = DataUtils.GetSqlConnection();
SqlCommand command = new SqlCommand("av_EventDetail_ws", connection);
command.CommandTimeout = Config.CommandTimeout;
command.CommandType = CommandType.StoredProcedure;
command.Parameters.Add("@evt_key", SqlDbType.Uniqueidentifier, 16).Value = evt_key;
System.Xml.XmlDataDocument xml = new System.Xml.XmlDataDocument();
try
{
System.Xml.XmlReader reader;
reader = command.ExecuteXmlReader();
xml.Load(reader);
}
catch (System.Data.SqlClient.SqlException ex)
{
throw ex;
}
catch (Exception ex)
{
throw ex;
}
finally
{
connection.Close();
}
try
{
XmlSerializer serializer = new XmlSerializer(typeof(EventList));
System.Xml.XmlNodeReader oReader = new System.Xml.XmlNodeReader(xml);
EventList = (EventList)serializer.Deserialize(oReader);
}
catch (Exception ex)
{
throw new Exception("Error:" + ex.Message);
}
return EventList;
}
iWeb Web Method setup
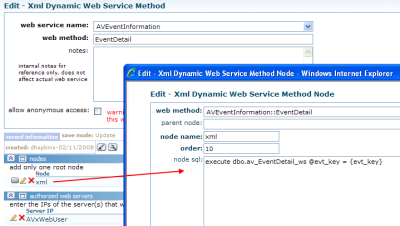
Add a Web Service in the Toolkit module of iWeb. Note how the DynamicXmlBuilder above links to this underlying metadata.
Web Service Code
Next, add ASP.NET Web Services Application called AUDC2008WS. Add references to:
- AUDC2008Web - the class library described above
- Common - Avectra.netForum.Common
- Data - Avectra.netForum.Data
- xWebClasses - Avectra.netForum.xWeb.xWebClasses
Add a ASMX called AUDCWS and enter the following code:
using System;
using System.Data;
using System.Web;
using System.Xml;
using System.Xml.Schema;
using System.Xml.Serialization;
using System.Collections;
using System.Web.Services;
using System.Web.Services.Protocols;
using System.Web.Services.Description;
using System.ComponentModel;
using Avectra.netForum.xWeb;
using Avectra.netForum.xWeb.xWebSecure;
using Avectra.netForum.Common;
namespace AUDC2008WS
{
/// <summary>
/// Summary description for AUDCWS
/// </summary>
[WebService(Namespace = "http://www.avectra.com/2005/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[ToolboxItem(false)]
public class AUDCWS : Avectra.netForum.xWeb.xWebSecure.xWebServiceClass
{
[WebMethod]
[SoapHeader("AuthToken", Direction = SoapHeaderDirection.InOut)]
[SoapDocumentMethod(Use = SoapBindingUse.Literal)]
[XmlInclude(typeof(AUDC2008WSClasses.Events))]
public AUDC2008WSClasses.EventList EventDetail(string evt_key)
{
/// Call xWeb's ValidateToken to ensure program authenticated!!!
ValidateToken();
// Instantiate EventList object for return
AUDC2008WSClasses.EventList EventList = new AUDC2008WSClasses.EventList();
try
{
EventList = AUDC2008WSClasses.Functions.EventDetail(evt_key, this.szUserName);
}
catch (Exception ex)
{
Avectra.netForum.xWeb.FacadeData.xWebException oSoapException = new FacadeData.xWebException(ex);
if (!(ex is SoapException))
Authentication.UpdateTokenWithFault(xNet.authenticationToken);
SoapException se = oSoapException.GenerateXWebException(Context.Request.Url.AbsoluteUri, xNet.NamespaceVersionInfo());
throw se;
}
return EventList;
}
}
}
Build the Web Service and deploy the AUDCWS.dll and AUDC2008WSClasses.dll to the /xweb/bin/ folder and the AUDCWS.asmx to the /xweb/secure/ folder.
Integrating Code
Here is C# code that calls this web service. This the kind of code the integrator would write to call the EventDetail web method above.
Assuming that a web reference has been added to the URL endpoint and named AUDCWebService then this code calls the web method and returns an EventList object:
using System;
using System.Collections.Generic;
using System.Collections;
using System.Text;
using System.Xml;
using System.Xml.Serialization;
using System.Web;
using System.IO;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Configuration;
using WebClasses.AUDCWebService;
namespace WebClasses
{
public class EventFunctions
{
/// <summary>
/// Returns Events from Web Service
/// </summary>
/// <param name="szevt_key">Event Key</param>
/// <returns></returns>
public static AUDCWebService.EventList EventList(string szevt_key)
{
// first, authenticate to xWeb
WebClasses.Properties.Settings settings = new WebClasses.Properties.Settings();
xweb.netForumXML xWeb = new xweb.netForumXML();
xWeb.Authenticate(settings.userName, settings.password);
// instantiate EventList for return
AUDCWebService.EventList eventList = new AUDCWebService.EventList();
// Setup xWeb AuthorizationToken, set the Token's value
// from the earlier authentication
AUDCWebService.AuthorizationToken audcToken = new AuthorizationToken();
audcToken.Token = xWeb.AuthorizationTokenValue.Token.ToString();
// Instantiate web service
AUDCWebService.AUDCWS ws = new AUDCWebService.AUDCWS();
// Pass the AuthorizationToken
ws.AuthorizationTokenValue = audcToken;
// Call the Web Service's EventDetail web method:
eventList = ws.EventDetail(szevt_key);
return eventList;
}
}
}
Here is code that calls this method and outputs the EventList return:
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using WebClasses;
using WebClasses.AUDCWebService;
namespace AUDC2008Web
{
public partial class EventDetailPage : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
string szEventKey = "C9F0B1E1-096A-4C25-817A-925A6F8B4B30";
if(Request.QueryString["EventKey"] != null)
szEventKey = Request.QueryString["EventKey"].ToString();
EventList eventList = WebClasses.EventFunctions.EventList(szEventKey);
foreach (Event oEvent in eventList.Events)
{
/// Information from main Event
Response.Write("<h1>" + oEvent.evt_title + "</h1>");
Response.Write("Start Date: " + oEvent.evt_start_date.ToShortDateString() + "<br />");
Response.Write("End Date: " + oEvent.evt_end_date.ToShortDateString() + "<br />");
/// loop through Sessions collection
foreach (Session s in oEvent.Sessions)
{
Response.Write("<li>Session: " + s.ses_title + "</li>");
}
}
}
}
}