xWeb: ExecuteMethod C#
This case study shows you how to write Visual Studio .NET C# to call the ExecuteMethod web method in xWeb.
Code
// instantiate xWeb object
// assumes you have added Web Reference to the
// netFORUM xWeb URL endpoint and named the
// web reference "xweb"
xweb.netForumXML xml = new xweb.netForumXML();
// Call Authenticate web method
xml.Authenticate(settings.userName, settings.password);
// Prepare for calling ExecuteMethod
// declare "parameters" array of "xweb.Parameter"
// the size (here it is "2") should be based on
// how many parameters you will pass
xweb.Parameter[] parameters = new xweb.Parameter[2];
// instantiate new Parameter and set Name and Value properties
xweb.Parameter p = new xweb.Parameter();
p.Name = "cst_key";
p.Value = "6ad3674d-1cfc-4c1d-8300-d9078927c562";
// Add this Parameter to the "parameters" array
parameters[0] = p;
// Add a 2nd parameter, set Name and Value
xweb.Parameter p2 = new xweb.Parameter();
p2.Name = "AsOfDate";
p2.Value = "02/02/2008";
// Add the second Parameter to the "parameters" array
parameters[1] = p2;
// Instantiate a blank XmlNode
System.Xml.XmlNode oNode;
// Call web method, put the return into the XmlNode
// Note that we are passing the "parameters" collection as the
// third parameter to the web method
oNode = xml.ExecuteMethod("AVWebService", "GetUserInfo", parameters);
Managing the Return
You can develop Classes that are modeled on the XML result from a ExecuteMethod query. Next, you can deserialize the XmlNode returned by the web method into your Object of your Class. You can now work with your Object in an object-oriented manner, instead of parsing the XML code directly.
XML Result
Suppose the ExecuteMethod response for a particular query looks like this. For this example the assumption is that <Customer> is a non-repeating node:
<Customers>
<Customer>
<cst_eml_address_dn>dhopkins@avectra.com</cst_eml_address_dn>
<interests>
<interestcode>
<itc_code>Accounting</itc_code>
</interestcode>
<interestcode>
<itc_code>ERP</itc_code>
</interestcode>
</interests>
</Customer>
</Customers>
C# Classes
In C#, you can create serializable classes patterned on the XML result. In order to deserialize properly, the class names should be the same names as the XmlNodes, and the class properties should be the same name as the XML Elements (e.g. <cst_eml_address_dn>
and <itc_code>). Observe that we have the following classes:
- Customers patterned on the <Customers> node.
- Customer patterned on the <Customer> node.
- interests patterned on the <interests> node.
- interestcode patterned on the <interestcode> node.
Observe also that :
- Customers contains a Customer property .
- Customer contains an interests property .
- interests is a Collection of interestcodes.
Here are the classes:
#region ExecuteMethod GetUserInfo Serialization classes
[Serializable]
public class Customers
{
public Customers()
{ }
public Customer Customer = new Customer();
}
[Serializable]
public class Customer
{
public Customer()
{ }
public string cst_eml_address_dn;
public interests interests = new interests();
}
[Serializable]
public class interestcode
{
public interestcode()
{ }
public string itc_code;
}
[Serializable]
public class interests : System.Collections.CollectionBase
{
public interestcode this[int nIndex]
{
get
{
return (interestcode)this.InnerList[nIndex];
}
}
public void Add(interestcode oParameter)
{
this.List.Add(oParameter);
}
}
#endregion
Deserialize XmlNode
Next, once you get your XmlNode response from ExecuteMethod, you can deserialize the XmlNode as shown below. Once the Object is loaded, then you can work with the result in an object-oriented way and not mess with XML:
// call xWeb ExecuteMethod and get response into XmlNode
System.Xml.XmlNode oNode;
oNode = xml.ExecuteMethod("AVWebService", "GetUserInfo", parameters);
// instantiate Customers object
Customers cust = new Customers();
// Deserialize XML into your object
XmlSerializer serializer = new XmlSerializer(typeof(Customers));
System.Xml.XmlNodeReader oReader = new System.Xml.XmlNodeReader(oNode);
cust = (Customers)serializer.Deserialize(oReader);
// Get properties from the object
string szUserName = cust.Customer.cst_eml_address_dn;
// Loop through interests in object
foreach(interestcode i in cust.Customer.interests)
{
string szGroupCode = i.itc_code;
}
Here is what this class will look like at run-time from a debugger after deserialization:
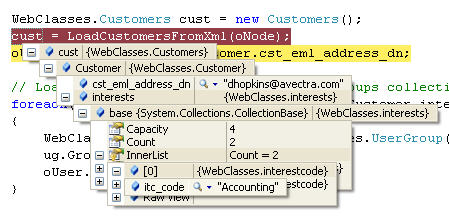
Considerations
Be sure you have using directives for:
using System.Xml;
using System.Xml.Serialization;
If you add extra fields to the ExecuteMethod query, then you will need to change your C# code accordingly.